Prototype Design Pattern
Prototype Design Pattern
When creating an object is time consuming and a costly affair and you already have a most similar object instance in hand, then you go for prototype pattern. Instead of going through a time consuming process to create a complex object, just copy the existing similar object and modify it according to your needs.
Its a simple and straight forward design pattern. Nothing much hidden beneath it. If you don’t have much experience with enterprise grade huge application, you may not have experience in creating a complex / time consuming instance. All you might have done is use the new operator or inject and instantiate.
If you are a beginner you might be wondering, why all the fuss about prototye design pattern and do we really need this design pattern? Just ignore, all the big guys requires it. For you, just understand the pattern and sleep over it. You may require it one day in future.
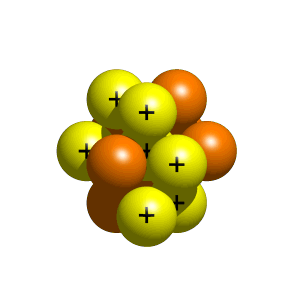
Prototype pattern may look similar to builder design pattern. There is a huge difference to it. If you remember, “the same construction process can create different representations” is the key in builder pattern. But not in the case of prototype pattern.
So, how to implement the prototype design pattern? You just have to copy the existing instance in hand. When you say copy in java, immediately cloning comes into picture. Thats why when you read about prototype pattern, all the literature invariably refers java cloning.
Simple way is, clone the existing instance in hand and then make the required update to the cloned instance so that you will get the object you need. Other way is, tweak the cloning method itself to suit your new object creation need. Therefore whenever you clone that object you will directly get the new object of desire without modifying the created object explicitly.
The prototype design pattern mandates that the instance which you are going to copy should provide the copying feature. It should not be done by an external utility or provider.
But the above, other way comes with a caution. If somebody who is not aware of your tweaking the clone business logic uses it, he will be in issue. Since what he has in hand is not the exact clone. You can go for a custom method which calls the clone internally and then modifies it according to the need. Which will be a better approach.
Always remember while using clone to copy, whether you need a shallow copy or deep copy. Decide based on your business needs. If you need a deep copy, you can use serialization as a hack to get the deep copy done. Using clone to copy is entirey a design decision while implementing the prototype design pattern. Clone is not a mandatory choice for prototype pattern.
In prototype pattern, you should always make sure that you are well knowledgeable about the data of the object that is to be cloned. Also make sure that instance allows you to make changes to the data. If not, after cloning you will not be able to make required changes to get the new required object.
Following sample java source code demonstrates the prototype pattern. I have a basic bike in hand with four gears. When I want to make a different object, an advance bike with six gears I copy the existing instance. Then make necessary modifications to the copied instance. Thus the prototype pattern is implemented. Example source code is just to demonstrate the design pattern, please don’t read too much out of it. I wanted to make things as simple as possible.
Sample Java Source Code for Prototype Design Pattern
package com.javapapers.sample.designpattern.prototype; class Bike implements Cloneable { private int gears; private String bikeType; private String model; public Bike() { bikeType = "Standard" ; model = "Leopard" ; gears = 4 ; } public Bike clone() { return new Bike(); } public void makeAdvanced() { bikeType = "Advanced" ; model = "Jaguar" ; gears = 6 ; } public String getModel(){ return model; } } public class Workshop { public Bike makeJaguar(Bike basicBike) { basicBike.makeAdvanced(); return basicBike; } public static void main(String args[]){ Bike bike = new Bike(); Bike basicBike = bike.clone(); Workshop workShop = new Workshop(); Bike advancedBike = workShop.makeJaguar(basicBike); System.out.println( "Prototype Design Pattern: " +advancedBike.getModel()); } }
|
Comments
Post a Comment
Please post comments here:-)